That's a good point. I almost never use monster groups, so that never occurred to me.minmay wrote:Pretty sure by "monster group" they meant a MonsterGroupComponent, not several different manually placed monsters.
It's kind of tricky to do... unless there is some undocumented feature for it; (which I don't know, but that you might

Here is one method; based on the earlier script: (It works with single and grouped monsters; or both at once.)
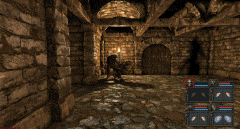
Code: Select all
--Script_entity
--List the monsters in the set here
groups = {}
set = {
"zarchton_1",
"zarchton_2",
"ratling_group1",
"ratling_group2",
"medusa_group1",
"medusa_group2",
"zarchton_3",
"zarchton_4",
}
for k,entry in pairs(set) do
if entry:match("group%d+") then
local monstergroup = findEntity(entry)
if monstergroup and monstergroup.monstergroup then
groups[#groups+1] = {["entry"] = k, ["id"] = monstergroup.id, ["mType"] = (monstergroup.monstergroup:getMonsterType()),
["count"] = monstergroup.monstergroup:getCount(), ["map"] = monstergroup.level,
["x"] = monstergroup.x, ["y"] = monstergroup.y, ["f"] = monstergroup.facing, ["e"] = monstergroup.elevation}
end
end
end
for x = #set, 1, -1 do
if set[x]:match("group%d+") then
table.remove(set, x)
end
end
--Adds onDie hooks
function _addHooks()
for x = 1, #set do
local monster = findEntity(set[x])
if monster and monster.monster then
monster.monster:addConnector("onDie", self.go.id, "deadCheck")
end
end
end
--Gets called by each monster as they die
function deadCheck(caller)
--No entries, means they are all dead
if #set < 1 then
dungeon_door_wooden_1.door:open() --========<< Triggered command/ opened door
set = {}
groups = {}
return
end
--removes the dying monster from the set
for k,v in pairs(set) do
if v == caller.go.id then
table.remove(set, k)
break
end
end
end
function _isInGroup(object)
local answer = false
for k, grp in pairs(groups) do
if object.level == grp.map and
object.x == grp.x and
object.y == grp.y and
object.elevation == grp.e then
answer = true
break
end
end
return answer
end
delayedCall(self.go.id, .1, "searchGroupMembers")
--Second Pass function:-----[called by delayedCall]------------
function searchGroupMembers()
for _,each in pairs(groups) do
for object in Dungeon.getMap(each['map']):allEntities() do
if object.monster then
if _isInGroup(object) then
set[#set+1] = object.id
_addHooks()
end
end
end
end
end
Code: Select all
--monsters.lua
-Groups MUST have "group" followed by a number in their name
--As in the examples group1, group2, group3
defineObject{
name = "medusa_group1",
baseObject = "medusa",
components = {
},
}
defineObject{
name = "medusa_group2",
baseObject = "medusa",
components = {
},
}
defineObject{
name = "ratling_group1",
baseObject = "ratling1",
components = {
},
}
defineObject{
name = "ratling_group2",
baseObject = "ratling1",
components = {
},
}
Demo Editor Project: https://www.dropbox.com/s/jlo17fxwmula5 ... d.zip?dl=0